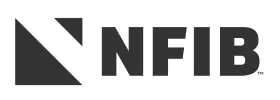
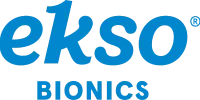

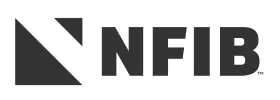
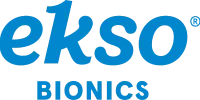

The Fast, Safe, and Reliable Way to Hire Elite Golang Developers in 48 Hours
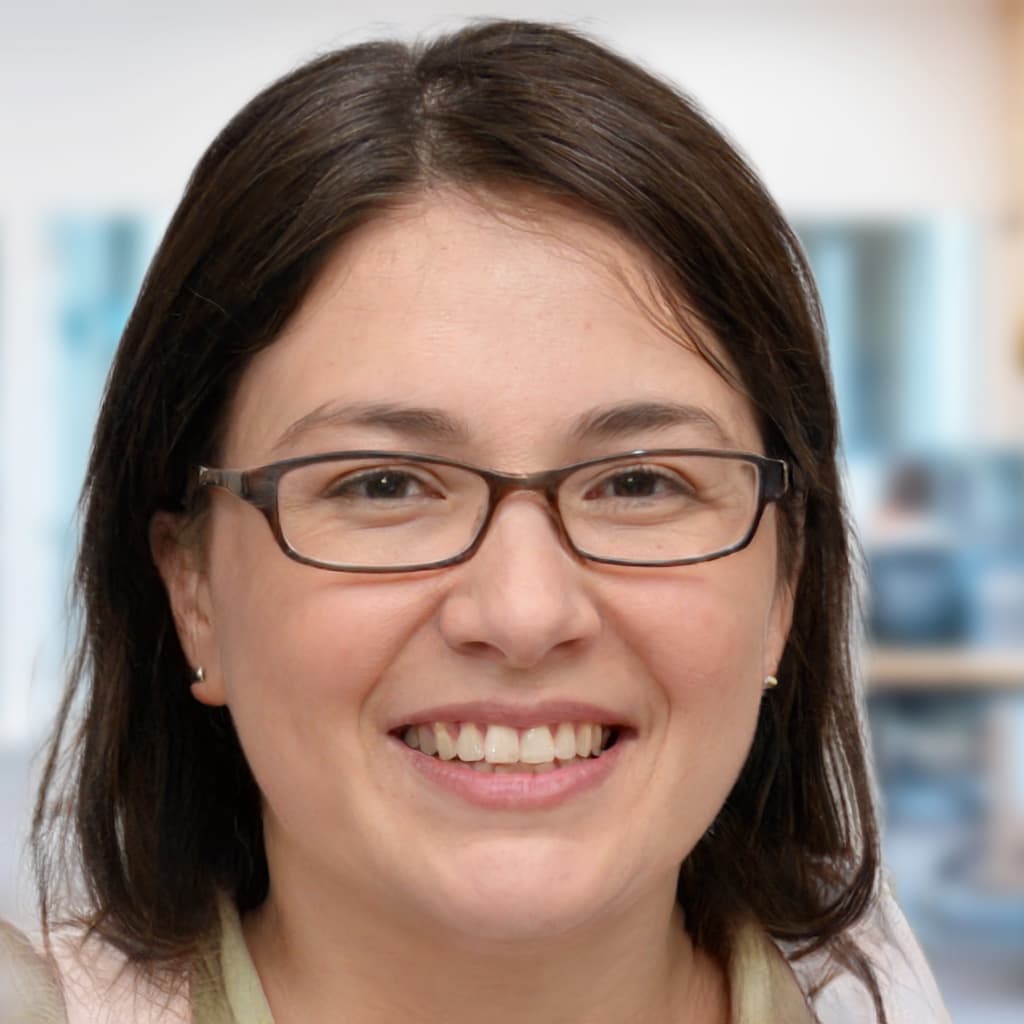

Build Amazing Development Teams
On Demand
Discover What Our Clients Have to Say About FullStack

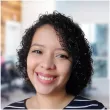
Book Talent Now
Frequently Asked Questions
Golang Hiring Guide
Introduction
As the popularity of the Go programming language continues to rise, more and more companies are looking to hire skilled Golang developers. However, finding the right candidate for the job can be challenging, especially if you are unfamiliar with the technical aspects of Golang development. FullStack has a wide range of developers available to join your team, but if you want to recruit directly, we have created this comprehensive Golang Developer Hiring Guide. Whether you are a hiring manager or a recruiter, this guide will help you navigate the hiring process and find the perfect Golang developer for your team. The guide is divided into four parts: Golang Conversational Interview Questions, Golang Technical Interview Questions, Golang Job Posting Template, and Golang Coding Challenge. Let's get started!
{{interview-qa-header="/hiring-docs/golang"}}
1. What inspired you to become a Golang developer?
I was drawn to the simplicity and efficiency of Golang. As a language designed for concurrent and scalable systems, it aligns well with my passion for building high-performance applications that can handle heavy traffic and large data sets.
2. Can you explain the difference between Go routines and threads?
In Go, a Goroutine is a lightweight thread managed by the Go runtime. Goroutines have their own stack and can be scheduled independently of other Goroutines, which makes them more efficient than traditional threads. They allow developers to write concurrent code that is easy to read and maintain.
3. How do you optimize Golang code for performance?
One way to optimize Golang code for performance is to minimize memory allocation by using pre-allocated slices and arrays. Another approach is to use channels and Goroutines to implement concurrency and avoid locking. Profiling and benchmarking tools can also help identify bottlenecks and improve performance.
4. Can you describe how you would implement a RESTful API using Golang?
I would use the net/http package in Golang to create the API endpoints and handle requests and responses. I would also leverage Golang's built-in support for JSON encoding and decoding to serialize and deserialize data. Authentication and authorization could be implemented using middleware and JWT tokens.
5. How do you handle errors in Golang?
In Golang, error handling is done using the error interface. I would use the defer statement to ensure that any resources are released properly, and I would also use panic and recover to handle unexpected errors and prevent crashes.
6. How do you test Golang code?
I would use the testing package in Golang to write unit tests, and I would also use tools like GoMock and GoStub to mock dependencies. Integration tests can be written using a tool like HTTParty or Selenium.
7. How do you handle concurrent access to shared resources in Golang?
I would use channels and locks to synchronize access to shared resources. For example, I would use a mutex to ensure that only one Goroutine can access a shared variable at a time. Alternatively, I would use channels to ensure that only one Goroutine can modify a data structure at a time.
8. Can you explain how garbage collection works in Golang?
Golang uses a concurrent garbage collector that runs in the background and automatically frees the memory that is no longer in use. It uses a mark-and-sweep algorithm to identify and collect unused memory, and it runs concurrently with the application code to minimize pauses.
9. How do you handle cross-site scripting (XSS) attacks in Golang?
I would use the html/template package in Golang to sanitize user input and prevent XSS attacks. This package automatically escapes HTML characters and ensures user input is displayed as plain text.
10. Can you describe your experience with Golang frameworks and libraries?
I have experience working with popular Golang frameworks like Gin and Echo, which provide robust tools for building web applications. I am also familiar with Golang libraries like GoMock and GoStub for testing and mocking, and I have used the Gorilla web toolkit for implementing websockets.
{{tech-qa-header="/hiring-docs/golang"}}
1. Write a function that takes a slice of integers and returns the sum of all the elements in the slice.
Answer:
<div style="padding-bottom: 2.85rem;"></div>
2. Implement a function that checks whether a given string is a palindrome or not.
Answer:
<div style="padding-bottom: 2.85rem;"></div>
3. Write a program that prints the Fibonacci sequence up to a given number n.
Answer:
<div style="padding-bottom: 2.85rem;"></div>
4. Implement a function that removes all duplicates from a slice of strings.
Answer:
<div style="padding-bottom: 2.85rem;"></div>
5. Write a program that reads a file and prints the number of lines, words, and characters in the file.
Answer:
{{job-qa-header="/hiring-docs/golang"}}
Introduction
Creating an excellent job posting is critical to attracting the best Golang developer candidates. In this guide, we'll provide tips for writing a job posting that accurately represents your company's culture and attracts qualified candidates.
<div style="padding-bottom: 2.85rem;"></div>
Job Title
The job title is the first thing that candidates will see, and it should accurately reflect the position's primary responsibilities. For a Golang developer role, the job title could be "Golang Developer," "Golang Software Engineer," or "Backend Golang Developer."
<div style="padding-bottom: 2.85rem;"></div>
Job Description
The job description should provide an overview of the job's key responsibilities and explain how the position fits into the company's overall goals. It should also include information about the company's culture, vision, and values.
<div style="padding-bottom: 1.14rem;"></div>
<span class="guide_indent-text">Example:</span>
<p span class="guide_indent-text">We seek a highly skilled Golang developer to join our team. In this role, you will develop and maintain high-performance, scalable, and reliable backend applications using Golang. You will work closely with other developers and stakeholders to design and implement software solutions that meet our business requirements. You should have a passion for programming and be familiar with agile software development practices.</p>
<div style="padding-bottom: 2.85rem;"></div>
Key Responsibilities
The key responsibilities section should provide a detailed overview of what the candidate will be doing on a day-to-day basis.
<div style="padding-bottom: 1.14rem;"></div>
<span class="guide_indent-text">Example:</span>
<ul><li>Develop high-performance, scalable, and reliable backend applications using Golang</li><li>Collaborate with other developers and stakeholders to design and implement software solutions</li><li>Write clean, maintainable, and efficient code</li><li>Optimize applications for maximum speed and scalability</li><li>Identify and resolve performance and scalability issues</li><li>Participate in code reviews and contribute to the development of best practices</li><li>Stay up-to-date with emerging trends and technologies in Golang and backend development</li></ul>
<div style="padding-bottom: 2.85rem;"></div>
Requirements
The requirements section should outline the skills and qualifications required to be considered for the position.
<div style="padding-bottom: 1.14rem;"></div>
<span class="guide_indent-text">Example:</span>
<ul><li>Bachelor's degree in Computer Science or a related field</li><li>Strong proficiency in Golang programming language</li><li>Experience developing high-performance, scalable, and reliable backend applications</li><li>Familiarity with agile software development practices</li><li>Strong problem-solving skills</li><li>Excellent communication and collaboration skills</li></ul>
<div style="padding-bottom: 2.85rem;"></div>
Preferred Qualifications
The preferred qualifications section should outline skills and experience that would be beneficial but not required for the position.
<div style="padding-bottom: 1.14rem;"></div>
<span class="guide_indent-text">Example:</span>
<ul><li>Experience with other programming languages such as Python or Java</li><li>Familiarity with cloud platforms such as AWS or GCP</li><li>Knowledge of containerization and orchestration technologies such as Docker and Kubernetes</li><li>Experience with test-driven development and automated testing frameworks</li><li>Familiarity with CI/CD pipelines and DevOps principles</li></ul>
<div style="padding-bottom: 2.85rem;"></div>
Benefits
The benefits section should outline the perks and benefits of the position. This includes health insurance, retirement plans, paid time off, flexible scheduling, and more. Highlighting your company's benefits can help attract top talent.
<span class="guide_indent-text">Example:</span>
<ul><li>Competitive salary and benefits package</li><li>Opportunity to work on exciting projects and cutting-edge technologies</li><li>Flexible working hours and remote work options</li><li>Health and wellness benefits, including gym memberships and wellness programs</li><li>Professional development opportunities, including conferences and training programs</li></ul>
<div style="padding-bottom: 2.85rem;"></div>
How to Apply
The how to apply section should provide clear instructions on how to apply for the job, including what materials to submit and where to send them.
<span class="guide_indent-text">Example:</span>
<p span class="guide_indent-text">To apply for this position, please send your resume, cover letter, and code samples to [insert email address]. Please also include your salary expectations and your earliest available start date. We will review your application and contact you if we decide to proceed with your candidacy.</p>
<div style="padding-bottom: 2.85rem;"></div>
Conclusion
A well-written job posting is critical to attracting the best candidates for your Golang developer position. Following the guidelines outlined in this guide, you can create a job posting that accurately reflects your company's culture, values, and goals while highlighting the key responsibilities and qualifications needed for the role. Remember to use clear and concise language, provide detailed information about the position, and make the application process easy for candidates. With the right job posting, you'll be able to attract the best Golang developers to your team and achieve your business goals.
{{challenge-qa-header="/hiring-docs/golang"}}
Challenge Instructions:
Implement a function in Golang that takes a string as input and returns a new string that is the reverse of the input string. Your function should also remove any vowels from the reversed string.
Answer:
<div style="padding-bottom: 2.85rem;"></div>
Conclusion
In conclusion, hiring a Golang developer can be a challenging task, but with the right approach and tools, it can be a smooth process. We hope this Golang Developer Hiring Guide has provided you with valuable insights and practical resources that you can use to identify the best Golang talent for your team. Remember to use the conversational and technical interview questions, the job posting template, and the coding challenge to create a comprehensive and effective hiring process. With these resources, you can confidently hire the right Golang developer to help take your company to the next level. Good luck!