1. Create React Native project
Assuming that you have the environment ready to create a project with React Native Cli, you’re going to use the next command to create an app with a template of typescript:
npx react-native init LottieApp --template react-native-template-typescript
To start metro, run the next command in React Native project folder:
npx react-native start
To start the app, run the next command in other terminal:
npx react-native run-ios / npx react-native run-android
2. Install Lottie library
To install the library, you have to install the dependencies and set up the environment for iOS and Android. In order to do it follow the following instructions:
a. Install lottie-react-native and lottie-ios (3.1.8):
npm i --save lottie-react-native
npm i --save lottie-ios@3.1.8
Then go to your iOS folder and run:
pod install
b. Now that you have installed the library and everything looks good, we have to import the library in the view that you want. In this tutorial we’re going to use our main view, App.tsx
Before using the library, you have to know how to use it and which components you have available, you can find this information in the API documentation.
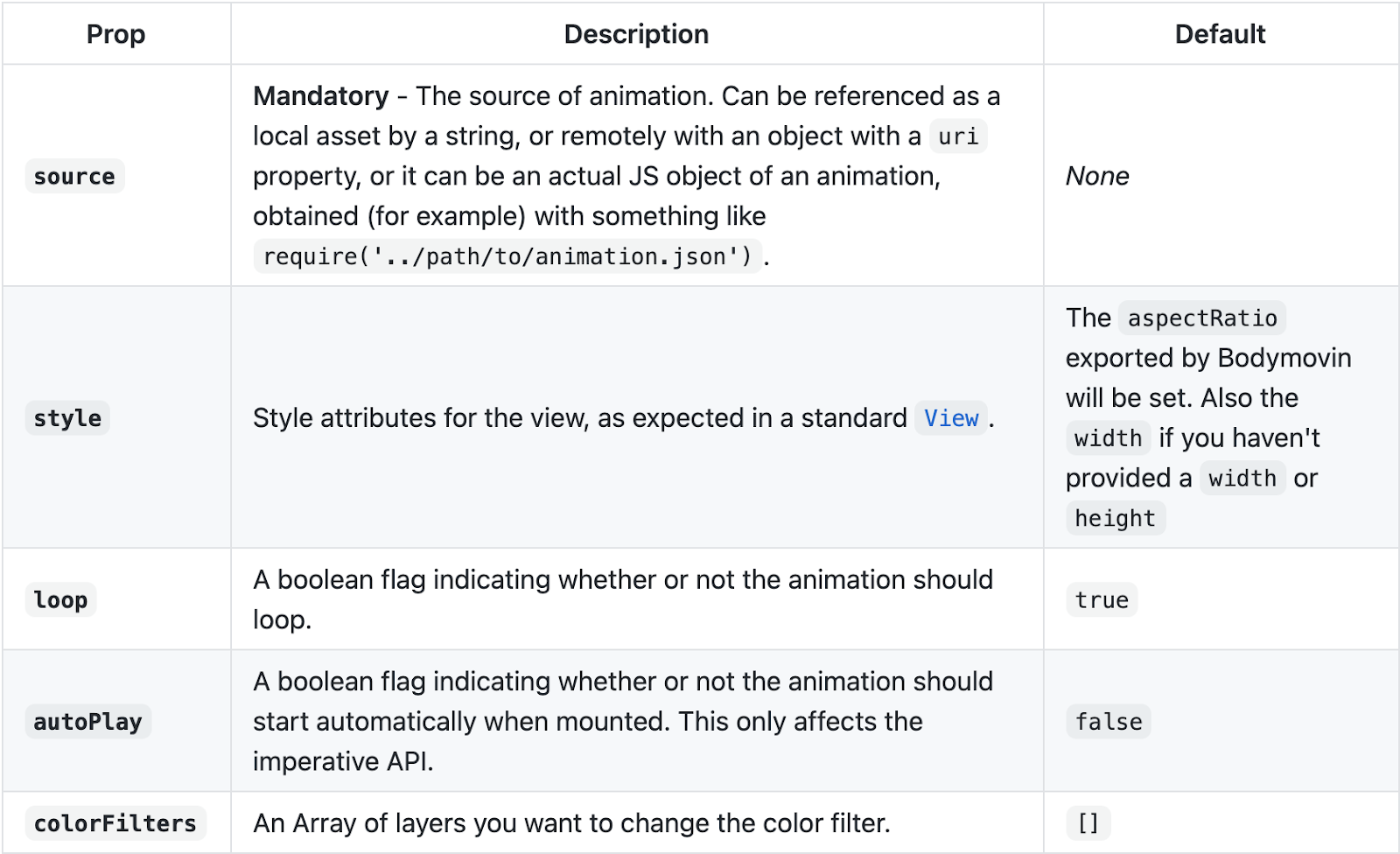
3. Create or download the Lottie example
You can create your own animation using Adobe After Effects animations exported as JSON with Bodymovin and then export it as JSON to be used in the project. Or you can download some examples from the official page LottieFiles.
For this tutorial we’re going to use an animation from the website Lottiefiles. Download this file as a Lottie JSON:
4. Add Lottie into the project
Now that we have our animation, move it to a new folder in the project. We’re going to move the file to the next route:
src/animations
Rename the file to software-development.json and save it.
5. Integrate the library into the project
To integrate this library you have to import it:
import LottieView from 'lottie-react-native';
And then to use this component, we just need to use it:
ref={(ref) => (animation = ref)}
source={require('./src/animations/software-development.json')}
/>
We’re going to make some changes in our file App.tsx to include this component and update the design of the screen.
/** * Sample React Native App * https://github.com/facebook/react-native * * Generated with the TypeScript template * https://github.com/react-native-community/react-native-template-typescript * * @format*/
import React, {useEffect} from 'react';
import {SafeAreaView, StyleSheet, View, Text, StatusBar} from 'react-native';
import {Colors} from 'react-native/Libraries/NewAppScreen';
import LottieView from 'lottie-react-native';
const App = () => {
let animation: any;
useEffect(() => animation.play());
return (
<>
<statusbar barstyle="dark-content"></statusbar>
<safeareaview style="{styles.safeArea}"></safeareaview>
<view style="{styles.sectionTitle}"></view>
<text style="{styles.title}">React Native & Lottie</text>
ref={(ref) => (animation = ref)} source={require('./src/animations/software-development.json')} />
</>
);
};
const styles = StyleSheet.create({
safeArea: {
flex: 1,
backgroundColor: Colors.white,
},
sectionTitle: {
position: 'absolute',
top: 100,
right: 0,
left: 0,
},
title: {
fontSize: 24,
fontWeight: '600',
color: Colors.black,
textAlign: 'center',
},
});
exportdefault App;
6. Enjoy it...

Using techniques like what is listed above, here at FullStack Labs we have had the opportunity to address our clients’ concerns and they love it! If you are interested in joining our team, please visit our Careers page.