“Mobile-First” has been a design philosophy for years now, but it’s becoming increasingly relevant as Google’s mobile-first indexing will look for a mobile version of a webpage before a desktop version to rank and index. However, with this change in indexing comes rules regarding mobile website code and best practices to ensure the best experience and SEO for your mobile site.
AMP (Accelerated Mobile Pages) positives and negatives
There are a number of frameworks that solve these problems and ensure that you are implementing best practices for your mobile experience. One of these that stands out for several reasons is Google’s AMP (Accelerated Mobile Pages). It’s not only a framework with components but also allows for rich content such as Web Stories that allow advertisements and blurbs to show throughout the web. However, if you are only concerned with creating a user-friendly mobile experience that is compliant with Google’s mobile-first indexing, then you have some options and alternatives to using AMP.
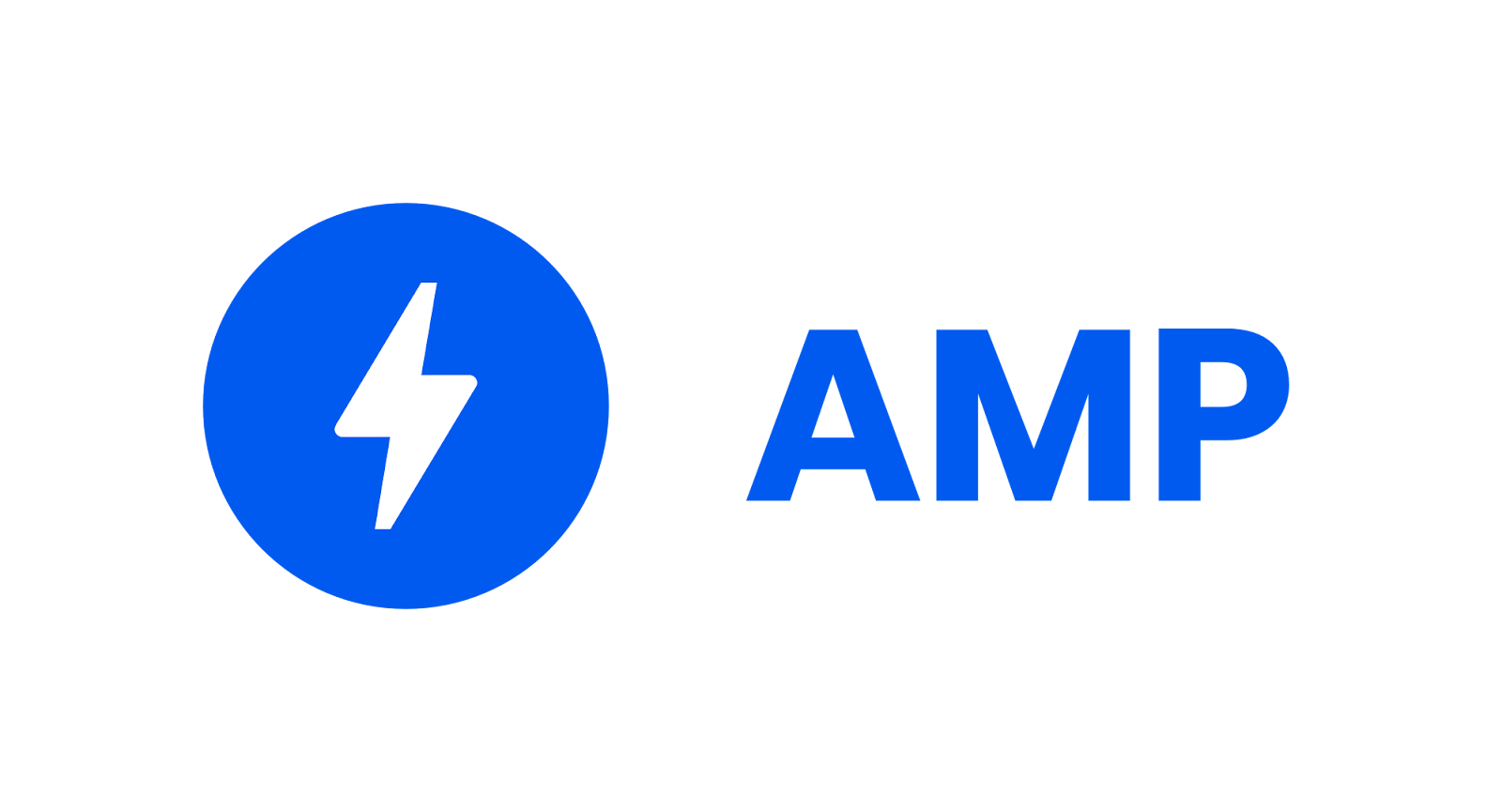
AMP excels at enforcing best practices for a website’s mobile experience. The main benefit of this is very high page speed loads. With more than 50% of users exiting a mobile website if it takes longer than 3 seconds to load, high load speeds are an integral part of a mobile experience.
One way AMP enforces this is by making sure all JavaScript is asynchronous and does not delay load time. Custom JavaScript can only be added if it is asynchronous, and third-party JavaScript must be added in an iframe. Another thing AMP enforces is making sure all CSS is inline. What this does is remove an HTTP call as well as making sure the developer limits his CSS to fit the maximum 50kb allowed for an inline style sheet. This affects web fonts as well, as they will load quicker with asynchronous JavaScript and zero requests for CSS files.
AMP also comes with a library of components that could be used for your mobile website that are lightweight and will automatically use best practices. One example of a component this is helpful for is a carousel, which might use a large amount of JavaScript as well as high-resolution images. AMP’s solution for this is to use its own components that enforce a static width and height, which increases load time for images, as well as asynchronous JavaScript functionality built-in. Below is an example of what an AMP component would look like in your code.
<amp-carousel type="slides"
width="500"
height="300"
controls
loop
autoplay
delay="3000" data-next-button-aria-label="Next"
data-previous-button-aria-label="Back"
role="region"
aria-label="Fullstack Labs Blog Carousel">
<amp-img src="https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/610491ddbbba72fd49590e96_How%20to%20Migrate%20a%20Website%20to%20Webflow.svg"
width="500"
height="300"></amp-img>
<amp-img src="https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/61049081905a8e0434053715_Reducing%20Process%20Complexity%20in%20Diagrams%20Using%20Archimate.svg"
width="500"
height="300"></amp-img>
<amp-img src="https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/60faf8f1931a2ebe109cc358_react%20hooks%20api.svg"
width="500"
height="300"></amp-img>
</amp-carousel>
It’s easy to use AMP and follow its standards because of the AMP Validator. When using AMP in your project, you will be able to paste your code into the validator (or use the Chrome Extension), and it will let you know if any code is violating any of the best practices. This is great because it takes out a lot of the trouble of debugging. However, AMP is very strict and you may not need to follow all of its rules for your website.
Nachos UI, an alternate approach
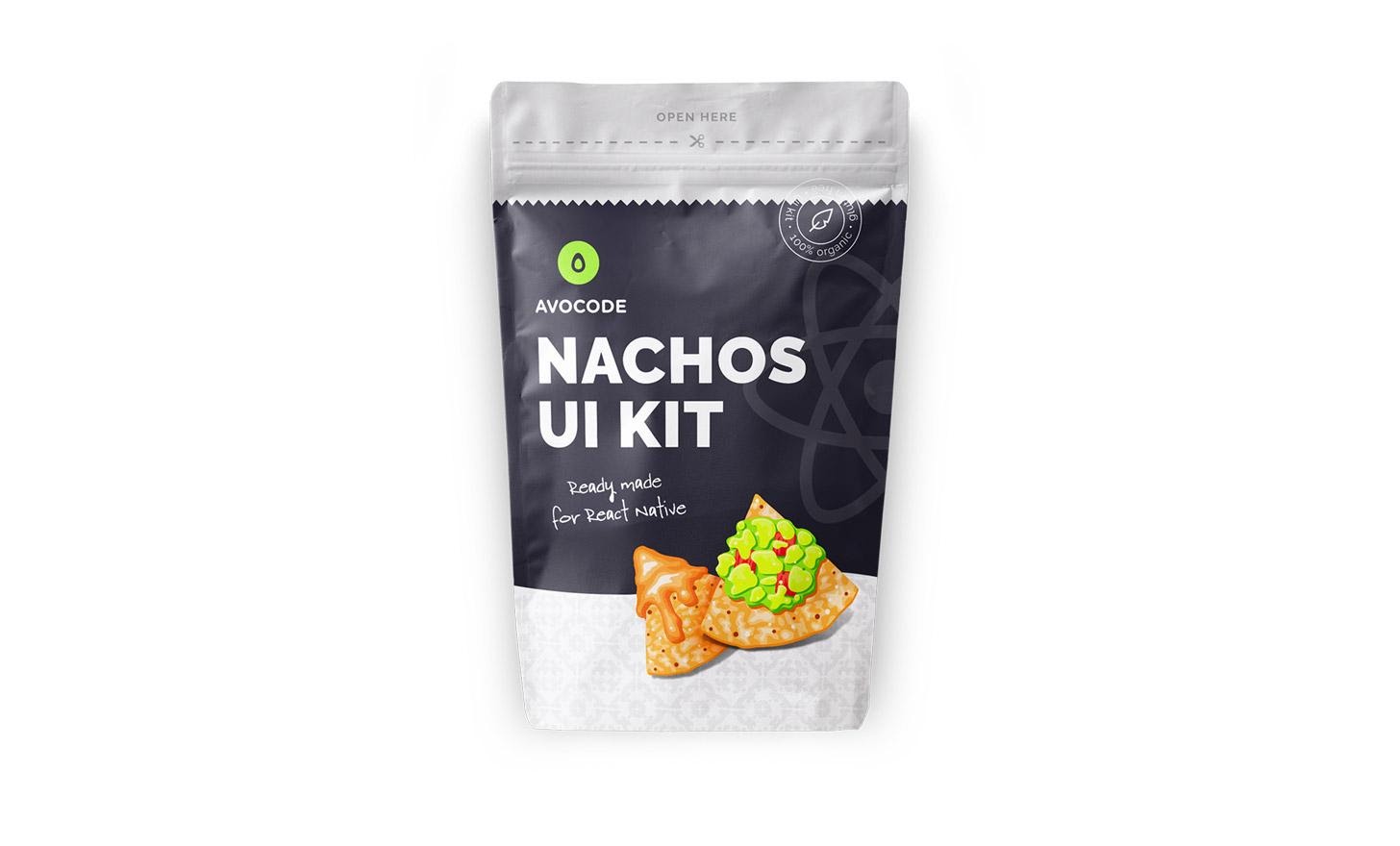
One framework that has a similar component library and design philosophy is Nachos UI by Avocode, which is used for React Native. Nachos UI allows for more freedom than AMP so it may be preferable depending on your use case. What it does is provide you with readymade components so that you can focus on other areas of optimization for your mobile experience. It will allow you to accomplish much more in a shorter time period while using approved, bug-free code. Below is an example of a carousel from Nachos UI.
const CarouselExample = () => {
return (
<View>
<H4>Example:</H4>
<View style={{ marginVertical: 15 }}>
<Carousel
images={[
'https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/610491ddbbba72fd49590e96_How%20to%20Migrate%20a%20Website%20to%20Webflow.svg',
'https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/61049081905a8e0434053715_Reducing%20Process%20Complexity%20in%20Diagrams%20Using%20Archimate.svg',
'https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/60faf8f1931a2ebe109cc358_react%20hooks%20api.svg'
]}
/>
</View>
</View>
)
}
MobileUI, a great experience for all devices
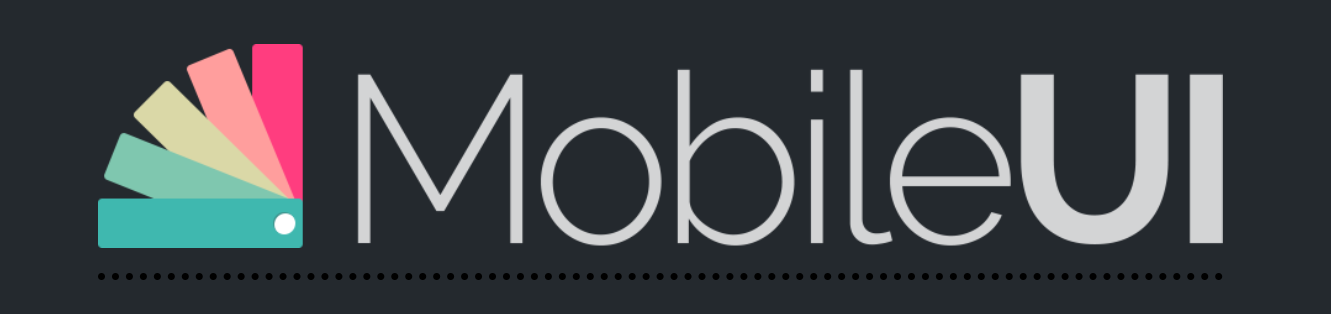
Another framework that I’ve found useful while building mobile experiences is MobileUI, which is a more opinionated and design-heavy framework. I find this framework to be very useful when you want your mobile app to have a very rich and modern design.
Its greatest benefit is that it has built-in functionality to give a different experience for Android and iOS users, which have many different built-in UI components. This way you can write the same code for all mobile users, and be confident that their experience is still native. Below is code for a swiping carousel, which will have different easing animations based on the operating system of the user.
<body class="grey-100"<
<div class="swiper-container swiper-gallery"<
<div class="swiper-wrapper"<
<div class="swiper-slide text-white align-center cover black-opacity-30 blend-soft-light" style="background-image:url(https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/610491ddbbba72fd49590e96_How%20to%20Migrate%20a%20Website%20to%20Webflow.svg)"<
</div<
<div class="swiper-slide text-white align-center cover black-opacity-30 blend-soft-light" style="background-image:url(https://assets-global.website-files.com/5d9bc5d562ffc22c37470958/61049081905a8e0434053715_Reducing%20Process%20Complexity%20in%20Diagrams%20Using%20Archimate.svg)"<
</div<
<div class="swiper-slide text-white align-center cover black-opacity-30 blend-soft-light" style="background-image:urlhttps://assets-global.website-files.com/5d9bc5d562ffc22c37470958/60faf8f1931a2ebe109cc358_react%20hooks%20api.svg)"<
</div<
</div<
</div<
<script type="text/javascript"<
new Swiper('.swiper-gallery', {
pagination: '.swiper-pagination'
});
</script<
</body<
I find this framework most helpful when I want to focus less on minimal page content and load speeds, and more on a rich experience with animations that will look good on all devices.
Custom approaches
Of course, another alternative to using AMP is to not use any framework at all. A Progressive Web Page (PWA) accomplishes the majority of what AMP strives for by ensuring best practices for mobile experiences, and experiences across all devices. There are many standards and techniques used that do not require a framework, with steps that can be taken to avoid any limitations that these frameworks might come with.
One technique that is very important for high load speeds is optimizing web fonts. I’ve personally found the font-display CSS property to be extremely useful for this.
@font-face { font-family: Arial; font-display: swap; }
Depending on your browser, using font-display “swap” will display the font in question immediately using a system font before switching to the font that it should be using. Since this does not work for all browsers, it should also be used in combination with the FontFaceObserver JavaScript library to see if your web font has been downloaded and is available yet. Then put in the code to check for the web font and switch your CSS to use it dynamically.
var font = new FontFaceObserver('Font Family', {
weight: 200
});
font.load().then(function () {
console.log('Font available');
}, function () {
console.log('Font unavailable');
});
Other important things that a web developer can make sure to implement so that they don’t need to rely on frameworks such as AMP include:
- Certain UX features, such as hiding popups from showing and turning popup containers into full-screen windows instead.
- Collapsible accordions rather than sidebars are also a practice that should be implemented.
- It is also important to link phone numbers and use the correct input types to create a native experience.
Loading JavaScript asynchronously as AMP does and embedding as much CSS as possible inline, as well as static image sizing and font optimization, would be the best ways to mimic a true AMP experience. AMP makes page load speeds instant to the eye, which increases user retention dramatically.
Conclusion
It’s becoming increasingly important to make sure your website has a fantastic mobile experience; often more so than desktop these days. As more users are browsing the web on their phones, and Google places a much bigger focus on mobile experiences for their indexing, a web developer must consider all of their options.
AMP has great SEO features and ensures best practices, but can be limiting. Nachos UI is a similar component library that offers much more freedom, but fewer tools for debugging. MobileUI also gives more freedom but has more opinionated and designed components and is less customizable than Nachos UI, but is better equipped to look native for different mobile devices. It’s up to a web developer to understand which framework might be best for them, or if they should forgo using a framework at all, in which case there is a large number of design practices they could follow.
These practices are changing fast as more and more data comes out on mobile web usage. While AMP, Nachos UI, and MobileUI stay continually updated and are therefore helpful in this regard, using your own best practices forces a web developer to stay up on the newest, freshest techniques. Ultimately, it is up to you which approach you want to take to develop your mobile site, but what is most important is to make sure it is looked after and treated with just as much--or more--care than a desktop site.